Available in versions: Dev (3.21) | Latest (3.20) | 3.19 | 3.18 | 3.17 | 3.16 | 3.15 | 3.14 | 3.13 | 3.12 | 3.11
Configuration and setup of the generator
Applies to ✅ Open Source Edition ✅ Express Edition ✅ Professional Edition ✅ Enterprise Edition
There are three binaries available with jOOQ, to be downloaded from https://www.jooq.org/download or from Maven central:
-
jooq-3.20.5.jar
The main library that you will include in your application to run jOOQ -
jooq-meta-3.20.5.jar
The utility that you will include in your build to navigate your database schema for code generation. This can be used as a schema crawler as well. -
jooq-codegen-3.20.5.jar
The utility that you will include in your build to generate your database schema
Configure jOOQ's code generator
You need to tell jOOQ some things about your database connection. Here's an example of how to do it for an Oracle database
<configuration> <!-- Configure the database connection here --> <jdbc> <driver>oracle.jdbc.OracleDriver</driver> <url>jdbc:oracle:thin:@[your jdbc connection parameters]</url> <user>[your database user]</user> <password>[your database password]</password> <!-- You can also pass user/password and other JDBC properties in the optional properties tag: --> <properties> <property><key>user</key><value>[db-user]</value></property> <property><key>password</key><value>[db-password]</value></property> </properties> </jdbc> <generator> <database> <!-- The database dialect from jooq-meta. Available dialects are named org.jooq.meta.[database].[database]Database. Natively supported values are: org.jooq.meta.ase.ASEDatabase org.jooq.meta.auroramysql.AuroraMySQLDatabase org.jooq.meta.aurorapostgres.AuroraPostgresDatabase org.jooq.meta.clickhouse.ClickHouseDatabase org.jooq.meta.cockroachdb.CockroachDBDatabase org.jooq.meta.databricks.DatabricksDatabase org.jooq.meta.db2.DB2Database org.jooq.meta.derby.DerbyDatabase org.jooq.meta.firebird.FirebirdDatabase org.jooq.meta.h2.H2Database org.jooq.meta.hana.HANADatabase org.jooq.meta.hsqldb.HSQLDBDatabase org.jooq.meta.ignite.IgniteDatabase org.jooq.meta.informix.InformixDatabase org.jooq.meta.ingres.IngresDatabase org.jooq.meta.mariadb.MariaDBDatabase org.jooq.meta.mysql.MySQLDatabase org.jooq.meta.oracle.OracleDatabase org.jooq.meta.postgres.PostgresDatabase org.jooq.meta.redshift.RedshiftDatabase org.jooq.meta.snowflake.SnowflakeDatabase org.jooq.meta.sqldatawarehouse.SQLDataWarehouseDatabase org.jooq.meta.sqlite.SQLiteDatabase org.jooq.meta.sqlserver.SQLServerDatabase org.jooq.meta.sybase.SybaseDatabase org.jooq.meta.teradata.TeradataDatabase org.jooq.meta.trino.TrinoDatabase org.jooq.meta.vertica.VerticaDatabase This value can be used to reverse-engineer generic JDBC DatabaseMetaData (e.g. for MS Access) org.jooq.meta.jdbc.JDBCDatabase This value can be used to reverse-engineer standard jOOQ-meta XML formats org.jooq.meta.xml.XMLDatabase This value can be used to reverse-engineer schemas defined by SQL files (requires jooq-meta-extensions dependency) org.jooq.meta.extensions.ddl.DDLDatabase This value can be used to reverse-engineer schemas defined by JPA annotated entities (requires jooq-meta-extensions-hibernate dependency) org.jooq.meta.extensions.jpa.JPADatabase This value can be used to reverse-engineer schemas defined by Liquibase migration files (requires jooq-meta-extensions-liquibase dependency) org.jooq.meta.extensions.liquibase.LiquibaseDatabase You can also provide your own org.jooq.meta.Database implementation here, if your database is currently not supported --> <name>org.jooq.meta.oracle.OracleDatabase</name> <!-- All elements that are generated from your schema (A Java regular expression. Use the pipe to separate several expressions) Watch out for case-sensitivity. Depending on your database, this might be important! You can create case-insensitive regular expressions using this syntax: (?i:expr) Whitespace is ignored and comments are possible. --> <includes>.*</includes> <!-- All elements that are excluded from your schema (A Java regular expression. Use the pipe to separate several expressions). Excludes match before includes, i.e. excludes have a higher priority --> <excludes> UNUSED_TABLE # This table (unqualified name) should not be generated | PREFIX_.* # Objects with a given prefix should not be generated | SECRET_SCHEMA\.SECRET_TABLE # This table (qualified name) should not be generated | SECRET_ROUTINE # This routine (unqualified name) ... </excludes> <!-- The schema that is used locally as a source for meta information. This could be your development schema or the production schema, etc This cannot be combined with the schemata element. If left empty, jOOQ will generate all available schemata. See the manual's next section to learn how to generate several schemata --> <inputSchema>[your database schema / owner / name]</inputSchema> </database> <!-- Generation flags: See advanced configuration properties --> <generate/> <target> <!-- The destination package of your generated classes (within the destination directory) jOOQ may append the schema name to this package if generating multiple schemas, e.g. org.jooq.your.packagename.schema1 org.jooq.your.packagename.schema2 --> <packageName>org.jooq.your.packagename</packageName> <!-- The destination directory of your generated classes --> <directory>/path/to/your/dir</directory> </target> </generator> </configuration>
See the configuration XSD, standalone code generation, and maven code generation for more details.
new org.jooq.meta.jaxb.Configuration() // Configure the database connection here .withJdbc(new Jdbc() .withDriver("oracle.jdbc.OracleDriver") .withUrl("jdbc:oracle:thin:@[your jdbc connection parameters]") .withUser("[your database user]") .withPassword("[your database password]") // You can also pass user/password and other JDBC properties in the optional properties tag: .withProperties( new Property() .withKey("user") .withValue("[db-user]"), new Property() .withKey("password") .withValue("[db-password]") ) ) .withGenerator(new Generator() .withDatabase(new Database() // The database dialect from jooq-meta. Available dialects are // named org.jooq.meta.[database].[database]Database. // // Natively supported values are: // // org.jooq.meta.ase.ASEDatabase // org.jooq.meta.auroramysql.AuroraMySQLDatabase // org.jooq.meta.aurorapostgres.AuroraPostgresDatabase // org.jooq.meta.clickhouse.ClickHouseDatabase // org.jooq.meta.cockroachdb.CockroachDBDatabase // org.jooq.meta.databricks.DatabricksDatabase // org.jooq.meta.db2.DB2Database // org.jooq.meta.derby.DerbyDatabase // org.jooq.meta.firebird.FirebirdDatabase // org.jooq.meta.h2.H2Database // org.jooq.meta.hana.HANADatabase // org.jooq.meta.hsqldb.HSQLDBDatabase // org.jooq.meta.ignite.IgniteDatabase // org.jooq.meta.informix.InformixDatabase // org.jooq.meta.ingres.IngresDatabase // org.jooq.meta.mariadb.MariaDBDatabase // org.jooq.meta.mysql.MySQLDatabase // org.jooq.meta.oracle.OracleDatabase // org.jooq.meta.postgres.PostgresDatabase // org.jooq.meta.redshift.RedshiftDatabase // org.jooq.meta.snowflake.SnowflakeDatabase // org.jooq.meta.sqldatawarehouse.SQLDataWarehouseDatabase // org.jooq.meta.sqlite.SQLiteDatabase // org.jooq.meta.sqlserver.SQLServerDatabase // org.jooq.meta.sybase.SybaseDatabase // org.jooq.meta.teradata.TeradataDatabase // org.jooq.meta.trino.TrinoDatabase // org.jooq.meta.vertica.VerticaDatabase // // This value can be used to reverse-engineer generic JDBC DatabaseMetaData (e.g. for MS Access) // // org.jooq.meta.jdbc.JDBCDatabase // // This value can be used to reverse-engineer standard jOOQ-meta XML formats // // org.jooq.meta.xml.XMLDatabase // // This value can be used to reverse-engineer schemas defined by SQL files // (requires jooq-meta-extensions dependency) // // org.jooq.meta.extensions.ddl.DDLDatabase // // This value can be used to reverse-engineer schemas defined by JPA annotated entities // (requires jooq-meta-extensions-hibernate dependency) // // org.jooq.meta.extensions.jpa.JPADatabase // // This value can be used to reverse-engineer schemas defined by Liquibase migration files // (requires jooq-meta-extensions-liquibase dependency) // // org.jooq.meta.extensions.liquibase.LiquibaseDatabase // // You can also provide your own org.jooq.meta.Database implementation // here, if your database is currently not supported .withName("org.jooq.meta.oracle.OracleDatabase") // All elements that are generated from your schema (A Java regular expression. // Use the pipe to separate several expressions) Watch out for // case-sensitivity. Depending on your database, this might be // important! // // You can create case-insensitive regular expressions using this syntax: (?i:expr) // // Whitespace is ignored and comments are possible. .withIncludes(".*") // All elements that are excluded from your schema (A Java regular expression. // Use the pipe to separate several expressions). Excludes match before // includes, i.e. excludes have a higher priority .withExcludes(""" UNUSED_TABLE # This table (unqualified name) should not be generated | PREFIX_.* # Objects with a given prefix should not be generated | SECRET_SCHEMA\.SECRET_TABLE # This table (qualified name) should not be generated | SECRET_ROUTINE # This routine (unqualified name) ... """) // The schema that is used locally as a source for meta information. // This could be your development schema or the production schema, etc // This cannot be combined with the schemata element. // // If left empty, jOOQ will generate all available schemata. See the // manual's next section to learn how to generate several schemata .withInputSchema("[your database schema / owner / name]") ) // Generation flags: See advanced configuration properties .withGenerate() .withTarget(new Target() // The destination package of your generated classes (within the // destination directory) // // jOOQ may append the schema name to this package if generating multiple schemas, // e.g. org.jooq.your.packagename.schema1 // org.jooq.your.packagename.schema2 .withPackageName("org.jooq.your.packagename") // The destination directory of your generated classes .withDirectory("/path/to/your/dir") ) )
See the configuration XSD and programmatic code generation for more details.
import org.jooq.meta.jaxb.* configuration { // Configure the database connection here jdbc { driver = "oracle.jdbc.OracleDriver" url = "jdbc:oracle:thin:@[your jdbc connection parameters]" user = "[your database user]" password = "[your database password]" // You can also pass user/password and other JDBC properties in the optional properties tag: properties { property { key = "user" value = "[db-user]" } property { key = "password" value = "[db-password]" } } } generator { database { // The database dialect from jooq-meta. Available dialects are // named org.jooq.meta.[database].[database]Database. // // Natively supported values are: // // org.jooq.meta.ase.ASEDatabase // org.jooq.meta.auroramysql.AuroraMySQLDatabase // org.jooq.meta.aurorapostgres.AuroraPostgresDatabase // org.jooq.meta.clickhouse.ClickHouseDatabase // org.jooq.meta.cockroachdb.CockroachDBDatabase // org.jooq.meta.databricks.DatabricksDatabase // org.jooq.meta.db2.DB2Database // org.jooq.meta.derby.DerbyDatabase // org.jooq.meta.firebird.FirebirdDatabase // org.jooq.meta.h2.H2Database // org.jooq.meta.hana.HANADatabase // org.jooq.meta.hsqldb.HSQLDBDatabase // org.jooq.meta.ignite.IgniteDatabase // org.jooq.meta.informix.InformixDatabase // org.jooq.meta.ingres.IngresDatabase // org.jooq.meta.mariadb.MariaDBDatabase // org.jooq.meta.mysql.MySQLDatabase // org.jooq.meta.oracle.OracleDatabase // org.jooq.meta.postgres.PostgresDatabase // org.jooq.meta.redshift.RedshiftDatabase // org.jooq.meta.snowflake.SnowflakeDatabase // org.jooq.meta.sqldatawarehouse.SQLDataWarehouseDatabase // org.jooq.meta.sqlite.SQLiteDatabase // org.jooq.meta.sqlserver.SQLServerDatabase // org.jooq.meta.sybase.SybaseDatabase // org.jooq.meta.teradata.TeradataDatabase // org.jooq.meta.trino.TrinoDatabase // org.jooq.meta.vertica.VerticaDatabase // // This value can be used to reverse-engineer generic JDBC DatabaseMetaData (e.g. for MS Access) // // org.jooq.meta.jdbc.JDBCDatabase // // This value can be used to reverse-engineer standard jOOQ-meta XML formats // // org.jooq.meta.xml.XMLDatabase // // This value can be used to reverse-engineer schemas defined by SQL files // (requires jooq-meta-extensions dependency) // // org.jooq.meta.extensions.ddl.DDLDatabase // // This value can be used to reverse-engineer schemas defined by JPA annotated entities // (requires jooq-meta-extensions-hibernate dependency) // // org.jooq.meta.extensions.jpa.JPADatabase // // This value can be used to reverse-engineer schemas defined by Liquibase migration files // (requires jooq-meta-extensions-liquibase dependency) // // org.jooq.meta.extensions.liquibase.LiquibaseDatabase // // You can also provide your own org.jooq.meta.Database implementation // here, if your database is currently not supported name = "org.jooq.meta.oracle.OracleDatabase" // All elements that are generated from your schema (A Java regular expression. // Use the pipe to separate several expressions) Watch out for // case-sensitivity. Depending on your database, this might be // important! // // You can create case-insensitive regular expressions using this syntax: (?i:expr) // // Whitespace is ignored and comments are possible. includes = ".*" // All elements that are excluded from your schema (A Java regular expression. // Use the pipe to separate several expressions). Excludes match before // includes, i.e. excludes have a higher priority excludes = """ UNUSED_TABLE # This table (unqualified name) should not be generated | PREFIX_.* # Objects with a given prefix should not be generated | SECRET_SCHEMA\.SECRET_TABLE # This table (qualified name) should not be generated | SECRET_ROUTINE # This routine (unqualified name) ... """ // The schema that is used locally as a source for meta information. // This could be your development schema or the production schema, etc // This cannot be combined with the schemata element. // // If left empty, jOOQ will generate all available schemata. See the // manual's next section to learn how to generate several schemata inputSchema = "[your database schema / owner / name]" } // Generation flags: See advanced configuration properties generate {} target { // The destination package of your generated classes (within the // destination directory) // // jOOQ may append the schema name to this package if generating multiple schemas, // e.g. org.jooq.your.packagename.schema1 // org.jooq.your.packagename.schema2 packageName = "org.jooq.your.packagename" // The destination directory of your generated classes directory = "/path/to/your/dir" } } }
See the configuration XSD and gradle code generation for more details.
configuration { // Configure the database connection here jdbc { driver = "oracle.jdbc.OracleDriver" url = "jdbc:oracle:thin:@[your jdbc connection parameters]" user = "[your database user]" password = "[your database password]" // You can also pass user/password and other JDBC properties in the optional properties tag: properties { property { key = "user" value = "[db-user]" } property { key = "password" value = "[db-password]" } } } generator { database { // The database dialect from jooq-meta. Available dialects are // named org.jooq.meta.[database].[database]Database. // // Natively supported values are: // // org.jooq.meta.ase.ASEDatabase // org.jooq.meta.auroramysql.AuroraMySQLDatabase // org.jooq.meta.aurorapostgres.AuroraPostgresDatabase // org.jooq.meta.clickhouse.ClickHouseDatabase // org.jooq.meta.cockroachdb.CockroachDBDatabase // org.jooq.meta.databricks.DatabricksDatabase // org.jooq.meta.db2.DB2Database // org.jooq.meta.derby.DerbyDatabase // org.jooq.meta.firebird.FirebirdDatabase // org.jooq.meta.h2.H2Database // org.jooq.meta.hana.HANADatabase // org.jooq.meta.hsqldb.HSQLDBDatabase // org.jooq.meta.ignite.IgniteDatabase // org.jooq.meta.informix.InformixDatabase // org.jooq.meta.ingres.IngresDatabase // org.jooq.meta.mariadb.MariaDBDatabase // org.jooq.meta.mysql.MySQLDatabase // org.jooq.meta.oracle.OracleDatabase // org.jooq.meta.postgres.PostgresDatabase // org.jooq.meta.redshift.RedshiftDatabase // org.jooq.meta.snowflake.SnowflakeDatabase // org.jooq.meta.sqldatawarehouse.SQLDataWarehouseDatabase // org.jooq.meta.sqlite.SQLiteDatabase // org.jooq.meta.sqlserver.SQLServerDatabase // org.jooq.meta.sybase.SybaseDatabase // org.jooq.meta.teradata.TeradataDatabase // org.jooq.meta.trino.TrinoDatabase // org.jooq.meta.vertica.VerticaDatabase // // This value can be used to reverse-engineer generic JDBC DatabaseMetaData (e.g. for MS Access) // // org.jooq.meta.jdbc.JDBCDatabase // // This value can be used to reverse-engineer standard jOOQ-meta XML formats // // org.jooq.meta.xml.XMLDatabase // // This value can be used to reverse-engineer schemas defined by SQL files // (requires jooq-meta-extensions dependency) // // org.jooq.meta.extensions.ddl.DDLDatabase // // This value can be used to reverse-engineer schemas defined by JPA annotated entities // (requires jooq-meta-extensions-hibernate dependency) // // org.jooq.meta.extensions.jpa.JPADatabase // // This value can be used to reverse-engineer schemas defined by Liquibase migration files // (requires jooq-meta-extensions-liquibase dependency) // // org.jooq.meta.extensions.liquibase.LiquibaseDatabase // // You can also provide your own org.jooq.meta.Database implementation // here, if your database is currently not supported name = "org.jooq.meta.oracle.OracleDatabase" // All elements that are generated from your schema (A Java regular expression. // Use the pipe to separate several expressions) Watch out for // case-sensitivity. Depending on your database, this might be // important! // // You can create case-insensitive regular expressions using this syntax: (?i:expr) // // Whitespace is ignored and comments are possible. includes = ".*" // All elements that are excluded from your schema (A Java regular expression. // Use the pipe to separate several expressions). Excludes match before // includes, i.e. excludes have a higher priority excludes = """ UNUSED_TABLE # This table (unqualified name) should not be generated | PREFIX_.* # Objects with a given prefix should not be generated | SECRET_SCHEMA\.SECRET_TABLE # This table (qualified name) should not be generated | SECRET_ROUTINE # This routine (unqualified name) ... """ // The schema that is used locally as a source for meta information. // This could be your development schema or the production schema, etc // This cannot be combined with the schemata element. // // If left empty, jOOQ will generate all available schemata. See the // manual's next section to learn how to generate several schemata inputSchema = "[your database schema / owner / name]" } // Generation flags: See advanced configuration properties generate {} target { // The destination package of your generated classes (within the // destination directory) // // jOOQ may append the schema name to this package if generating multiple schemas, // e.g. org.jooq.your.packagename.schema1 // org.jooq.your.packagename.schema2 packageName = "org.jooq.your.packagename" // The destination directory of your generated classes directory = "/path/to/your/dir" } } }
See the configuration XSD and gradle code generation for more details.
// The jOOQ-codegen-gradle plugin has been introduced in version 3.19. // Please use the official plugin instead of the third party plugin that was recommended before.
There are also lots of advanced configuration parameters, which will be treated in the manual's section about advanced code generation features. Note, you can find the official XSD file for a formal specification at:
https://www.jooq.org/xsd/jooq-codegen-3.20.1.xsd
Run jOOQ code generation
Code generation works by calling this class with the above property file as argument.
org.jooq.codegen.GenerationTool /jooq-config.xml
Be sure that these elements are located on the classpath:
- jooq-3.20.5.jar, jooq-meta-3.20.5.jar, jooq-codegen-3.20.5.jar, reactive-streams-1.0.3.jar, r2dbc-spi-1.0.0.RELEASE.jar
- The JDBC driver you configured
A command-line example (for Windows, OSX/Linux/etc. will be similar)
- Put the XML configuration file, jooq*.jar and the JDBC driver into a directory, e.g. C:\temp\jooq
- Go to C:\temp\jooq
- Run
java -cp jooq-3.20.5.jar;jooq-meta-3.20.5.jar;jooq-codegen-3.20.5.jar;reactive-streams-1.0.3.jar;r2dbc-spi-1.0.0.RELEASE.jar;[JDBC-driver].jar org.jooq.codegen.GenerationTool <XML-file>
Note that the XML configuration file can also be loaded from the classpath, in which case the path should be given as /path/to/my-configuration.xml
Run code generation from Eclipse
Of course, you can also run code generation from your IDE. In Eclipse, set up a project like this. Note that:
- this example uses jOOQ's log4j support by adding log4j.xml and log4j.jar to the project classpath.
- the actual jooq-3.20.5.jar, jooq-meta-3.20.5.jar, jooq-codegen-3.20.5.jar artefacts may contain version numbers in the file names.
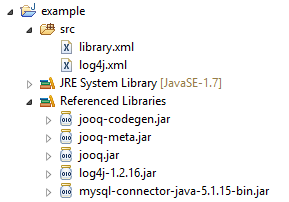
Once the project is set up correctly with all required artefacts on the classpath, you can configure an Eclipse Run Configuration for org.jooq.codegen.GenerationTool.
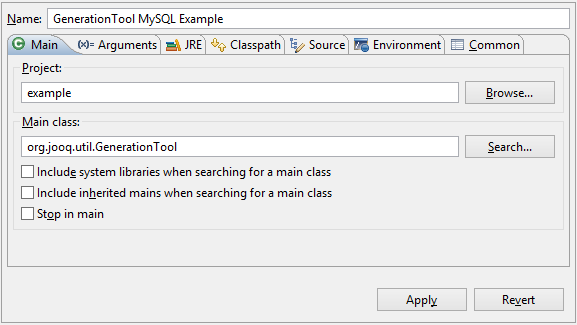
With the XML file as an argument
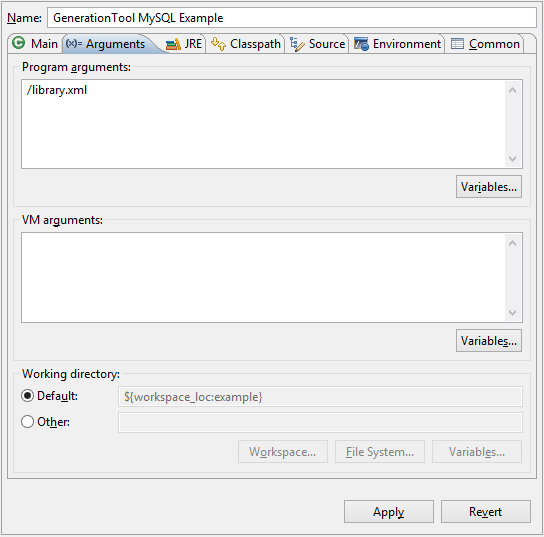
And the classpath set up correctly
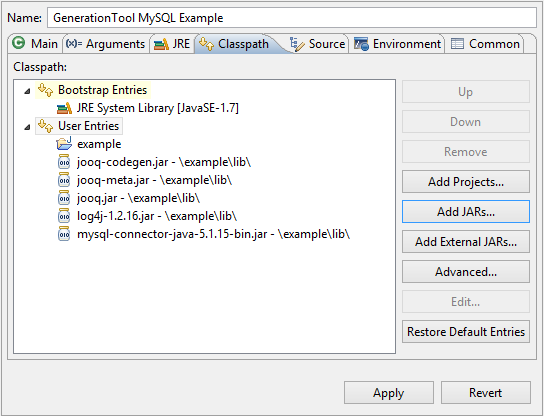
Finally, run the code generation and see your generated artefacts
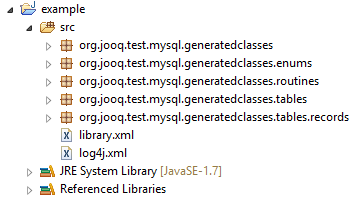
Integrate generation with Maven
Using the official jOOQ-codegen-maven plugin, you can integrate source code generation in your Maven build process:
<plugin> <!-- Specify the maven code generator plugin --> <!-- Use org.jooq for the Open Source Edition org.jooq.pro for commercial editions with Java 17 support, org.jooq.pro-java-11 for commercial editions with Java 11 support, org.jooq.pro-java-8 for commercial editions with Java 8 support, org.jooq.trial for the free trial edition with Java 17 support, org.jooq.trial-java-11 for the free trial edition with Java 11 support, org.jooq.trial-java-8 for the free trial edition with Java 8 support Note: Only the Open Source Edition is hosted on Maven Central. Install the others locally using the provided scripts, or access them from here: https://repo.jooq.org --> <groupId>org.jooq</groupId> <artifactId>jooq-codegen-maven</artifactId> <version>3.20.5</version> <!-- The plugin should hook into the generate goal --> <executions> <execution> <goals> <goal>generate</goal> </goals> </execution> </executions> <!-- Manage the plugin's dependency. In this example, we'll use a PostgreSQL database --> <dependencies> <dependency> <groupId>org.postgresql</groupId> <artifactId>postgresql</artifactId> <version>42.7.3</version> </dependency> </dependencies> <!-- Specify the plugin configuration. The configuration format is the same as for the standalone code generator --> <configuration> <!-- JDBC connection parameters --> <jdbc> <driver>org.postgresql.Driver</driver> <url>jdbc:postgresql:postgres</url> <user>postgres</user> <password>test</password> </jdbc> <!-- Generator parameters --> <generator> <database> <name>org.jooq.meta.postgres.PostgresDatabase</name> <includes>.*</includes> <excludes></excludes> <!-- In case your database supports catalogs, e.g. SQL Server: <inputCatalog>public</inputCatalog> --> <inputSchema>public</inputSchema> </database> <target> <packageName>org.jooq.codegen.maven.example</packageName> <directory>target/generated-sources/jooq</directory> </target> </generator> </configuration> </plugin>
Use jOOQ generated classes in your application
Be sure, both jooq-3.20.5.jar and your generated package (see configuration) are located on your classpath. Once this is done, you can execute SQL statements with your generated classes.
Feedback
Do you have any feedback about this page? We'd love to hear it!